Key Programming Practices for Modern Tech Development
In the realm of modern tech development, the application of best practices is not just beneficial but necessary for creating solid, efficient, and maintainable software.
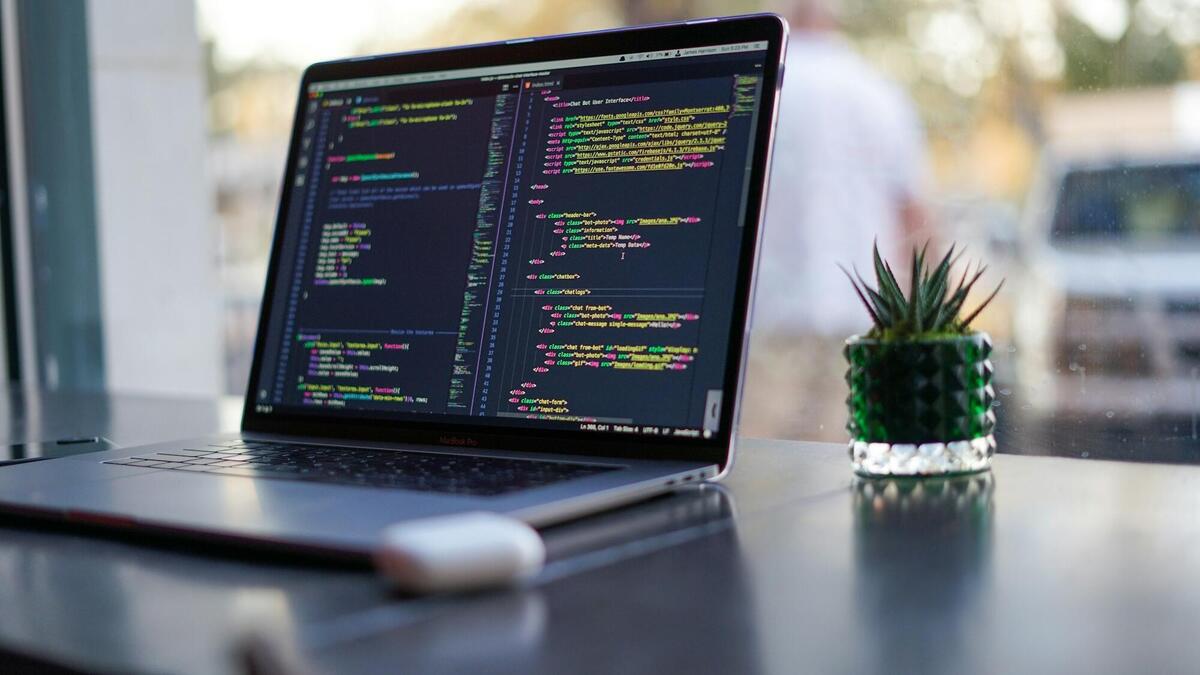
These practices not only streamline the development process but also enhance the quality of the final product, making it vital for developers to stay updated and adapt to these evolving standards.
Javascript in Modern Development
As a core technology of the web, JavaScript's significance in modern software development cannot be overstated.
It powers interactive web pages and is integral to front-end development, but its role extends to server-side technologies and even mobile app development.
Embracing best practices in JavaScript is the cornerstone for building scalable and efficient applications.
Javascript Date and Time Handling
Handling date and time is a fundamental aspect of programming that can become complex without proper techniques.
JavaScript offers built-in objects like Date for managing dates and times, but knowing how to use these tools effectively is key to ensuring your applications run smoothly across different locales and time zones.
- Best Practices For Date And Time In Javascript: To manage date and time efficiently in JavaScript, developers should utilize the Date object for creating, manipulating, and formatting dates and times. Libraries such as Moment.js or date-fns can help handle more complex date-time calculations and conversions, especially when dealing with different time zones or formats.
- Common Challenges And Solutions: One common challenge in handling dates and times in JavaScript is dealing with time zones and daylight saving time changes. Using libraries like Moment.js or the newer Luxon can help mitigate these issues by simplifying date-time calculations and conversions across time zones.
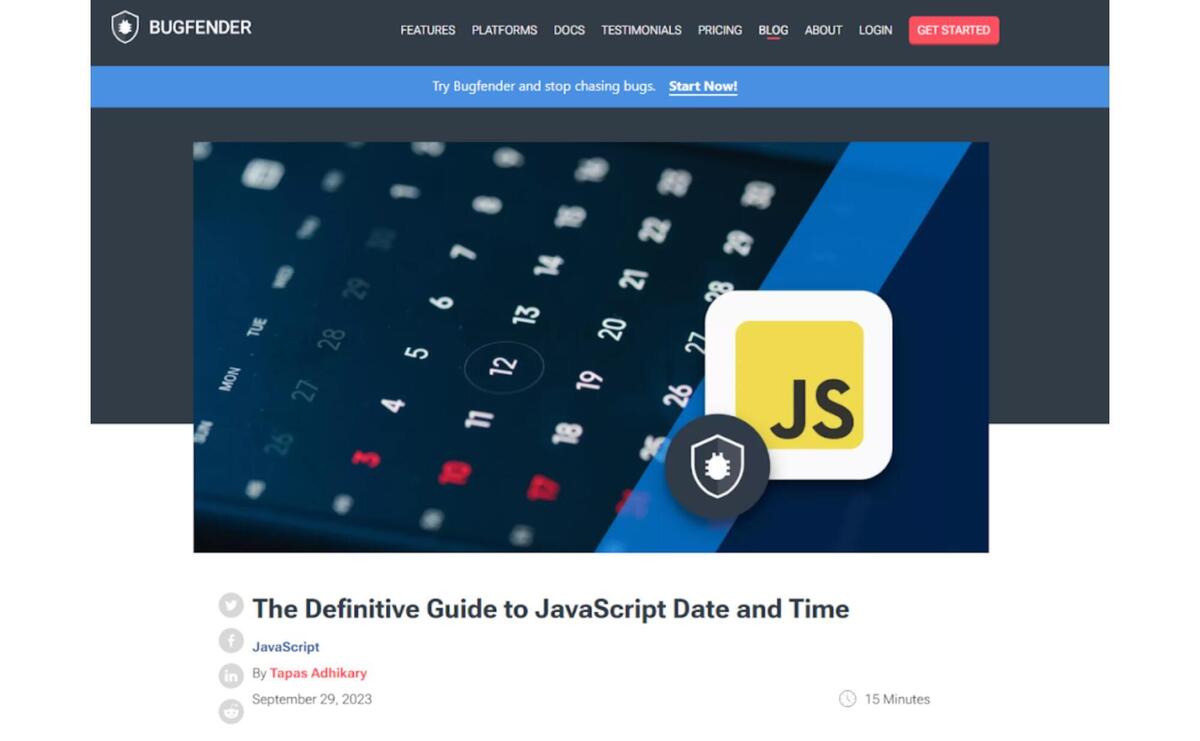
Structuring Javascript Code
- Modularization: As applications grow, maintaining a clean and manageable codebase becomes challenging. Structuring JavaScript code in a modular fashion, using patterns like modules or JavaScript frameworks (e.g., React, Angular), can greatly improve scalability and maintainability.
- Asynchronous Programming: JavaScript heavily relies on asynchronous programming patterns, especially in web environments. Mastering promises and async/await syntax is paramount for handling operations like API calls or any tasks that require waiting for operations to complete without blocking the main thread.
Performance Optimization in Javascript
- Efficiency Best Practices: To optimize JavaScript performance, developers should focus on minimizing reflows and repaints, compressing files, and lazy loading resources. Tools like Google's Lighthouse can be instrumental in identifying performance bottlenecks and providing actionable recommendations.
- Memory Management: Effective memory management is the first step to prevent leaks and ensure that applications perform well. Techniques such as avoiding global variables, using let and const properly, and being cautious with references to objects can help manage memory more effectively in JavaScript applications
Embracing Version Control Systems in Modern Software Development
Version control is indispensable in modern software development, acting as the backbone for managing changes and enabling team collaboration.
By understanding and implementing best practices in version control systems (VCS), developers can enhance productivity, ensure code integrity, and effectively manage both small and large projects.
Establishing Strong Commit Practices
Effective commit practices are integral for maintaining a clear and useful history in version control systems.
They enhance collaboration and ensure that changes can be tracked and understood by anyone within the team.
- Descriptive Commit Messages: It’s vital to write clear and descriptive commit messages. A well-crafted message should explain why a change was made, which can be very important for historical understanding and troubleshooting. Including references to issue or ticket numbers can also provide context and improve traceability.
- Atomic Commits: Each commit should represent a single logical change. This practice makes it easier to identify where bugs were introduced and simplifies the process of rolling back changes if something goes wrong.
- Avoid Half-Done Commits: Ensure that the code committed is complete and functional to avoid breaking the build. This practice not only keeps the development process smooth but also respects the time and effort of the entire team who may rely on your commits.
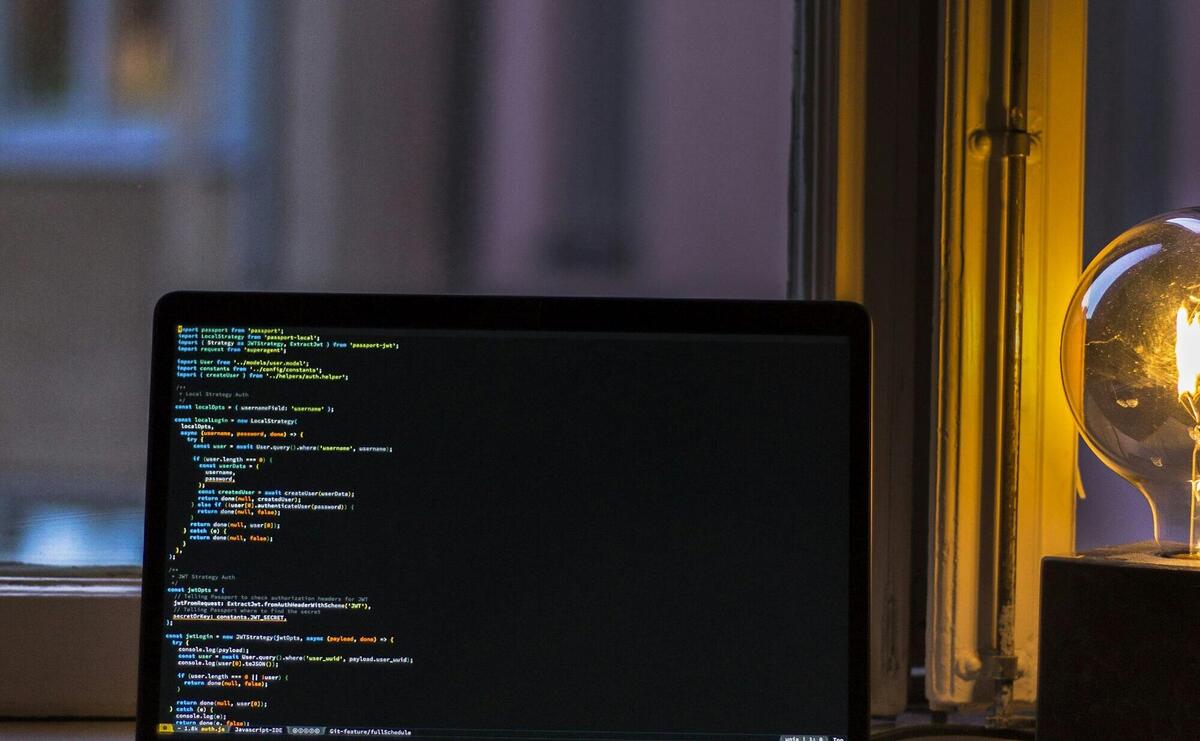
Optimizing Branch Management
- Clear Naming Conventions: Utilizing a clear and consistent naming convention for branches can prevent confusion and streamline workflow. Naming branches according to their purposes, such as feature/, bugfix/, or release/, helps in identifying their roles and managing them effectively.
- Pruning Branches: Regularly merging and deleting old branches that are no longer needed helps in maintaining a clean and efficient repository. This not only simplifies navigation but also minimizes the risk of clutter and confusion.
- Use Branches Strategically: Branches are powerful tools for managing features, fixes, and experiments. They allow developers to work independently on changes without affecting the stable main codebase. This separation aids in managing project complexity and aligns with continuous integration practices.
Enhancing Collaboration Through Pull Requests
- Pull Request Reviews: Before merging changes into the main branch, use pull requests to facilitate code reviews. This process ensures that at least one other team member reviews the changes, which enhances code quality and reduces the risk of bugs.
- Constructive Feedback In Reviews: Encourage a culture of constructive feedback during pull requests. Effective reviews should address potential bugs, architectural concerns, and performance issues. They should also provide an opportunity for team learning and improvement.
- Early Feedback With Draft Pull Requests: Utilizing draft pull requests allows developers to seek early feedback on their work without the pressure of finality. This can lead to more collaborative and iterative development.
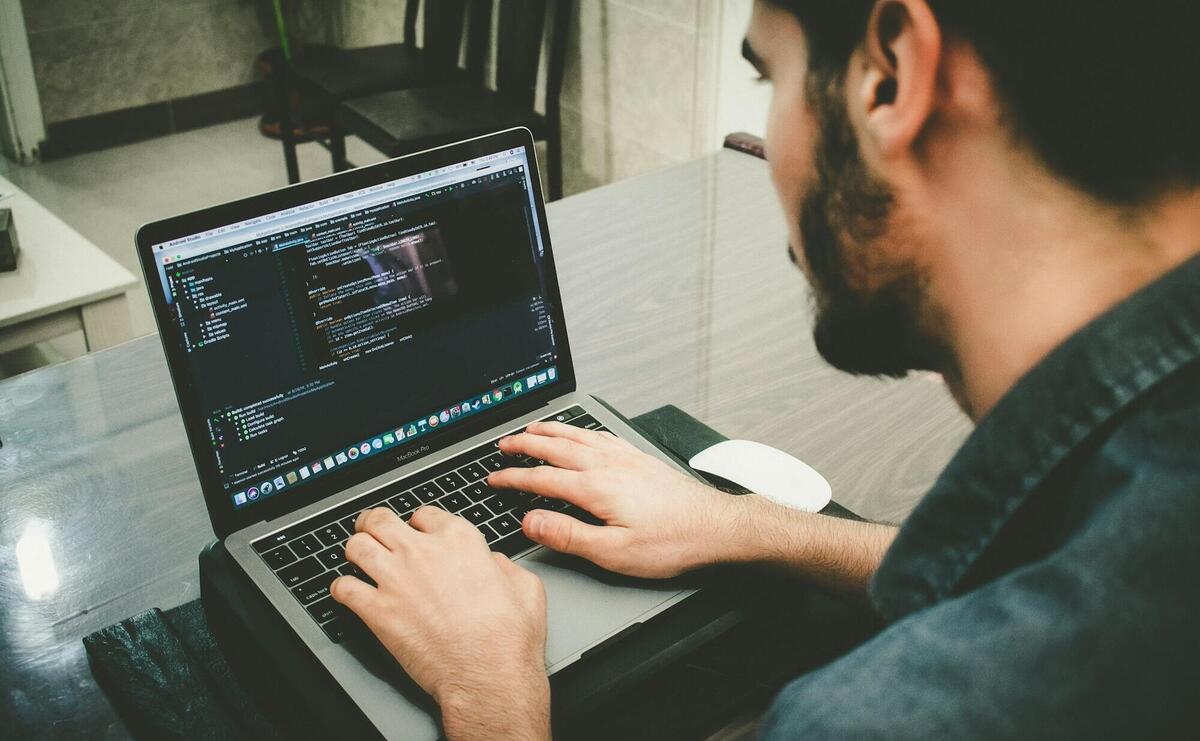
Enhancing Software Quality with Effective Code Reviews
Code reviews are a pivotal aspect of software development, serving not only to enhance code quality but also to foster team collaboration and learning.
Implementing code review practices can significantly reduce bugs and improve the functionality of software.
Streamlining the Review Process
A streamlined code review process ensures that reviews are both efficient and effective, focusing on significant issues without getting bogged down by trivialities.
- Clear Guidelines And Checklists: Establish a code review checklist to guide reviewers on what to look for, including code security, architecture, and test coverage. This structured approach helps in maintaining high standards across all reviews.
- Limit Review Scope: To maintain focus and effectiveness, limit the amount of code reviewed at any one time. Research suggests that beyond 200-400 lines, a reviewer's ability to spot defects diminishes. Keeping reviews concise ensures higher attention to detail.
- Use Metrics To Track Efficiency: Implementing metrics such as inspection rate, defect rate, and defect density can help assess and improve the efficiency of the code review process. These metrics aid in identifying problem areas and gauging the effectiveness of reviews.
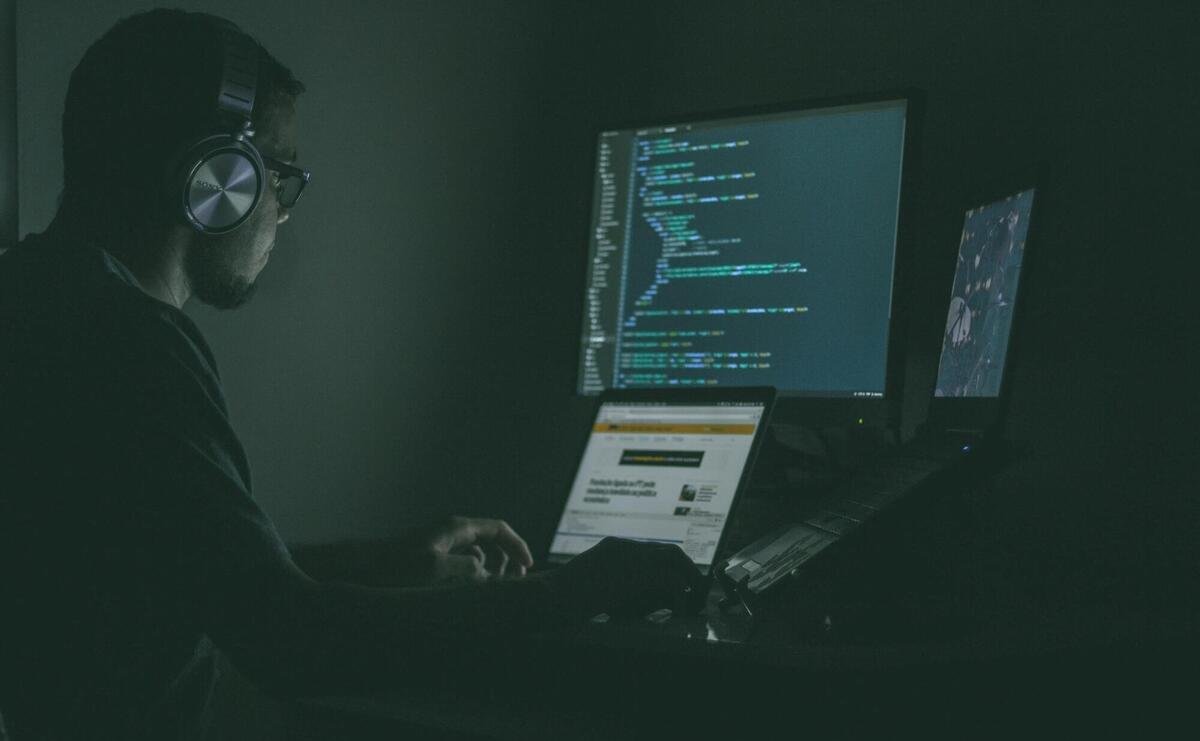
Fostering Constructive Feedback
- Cultivate A Positive Feedback Culture: Encourage feedback that is specific, actionable, and, most importantly, kind. Constructive feedback helps in building a positive team atmosphere and enhances the learning experience for all members involved.
- Address Non-Critical Comments Appropriately: Differentiate between important feedback and minor 'nitpicks.' Using prefixes like "nit:" can help in distinguishing less critical comments from those that are noteworthy. This helps in reducing frustration and focusing on more significant improvements during reviews.
- Regular And Empathetic Engagement: Especially for new joiners, ensure that the review process is welcoming and educational. This can be achieved by balancing rigor with understanding, providing guidance on the team’s coding standards, and encouraging questions.
Leveraging Automation and Tools
- Automate Where Possible: Incorporate tools that automatically check for common errors before the review stage. Tools like linters or static analysis can handle trivial issues, allowing reviewers to focus on more complex problems like logic errors or design improvements.
- Continuous Integration For Efficient Reviews: Utilize continuous integration tools to automate testing and ensure that pull requests are small and manageable. This practice helps in maintaining a smooth workflow and allows for quicker integration of changes.
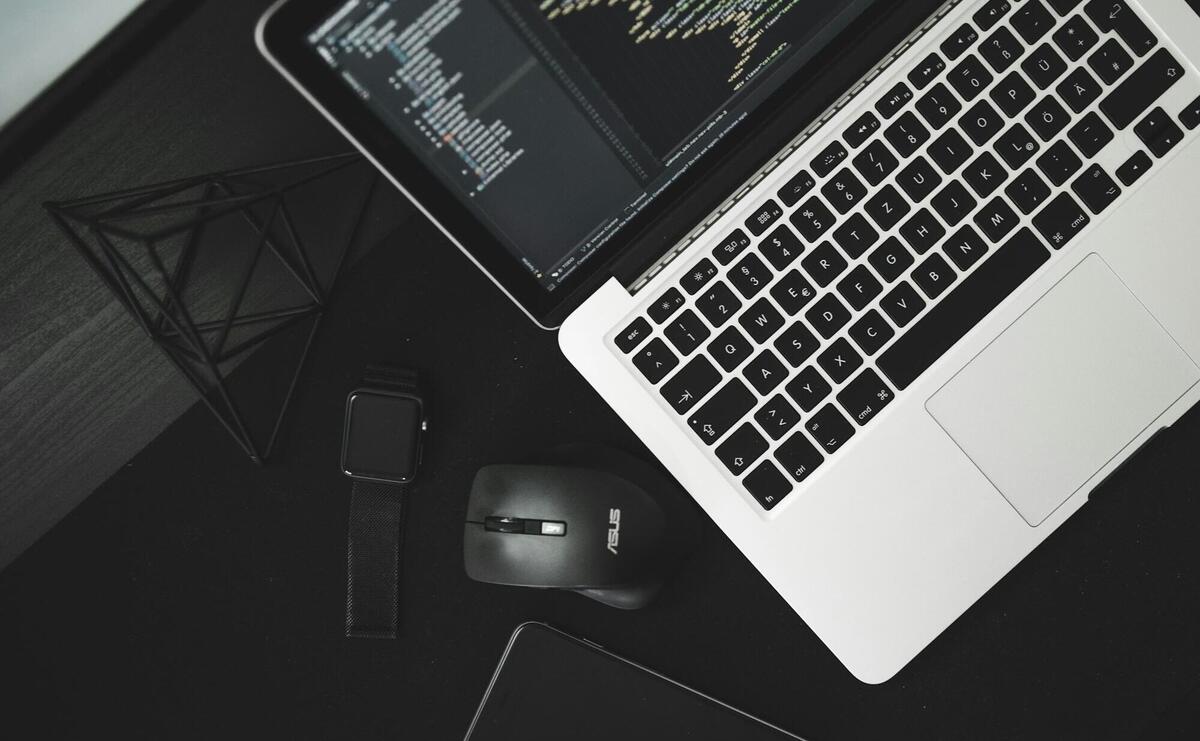
Endnote
In the dynamic realm of tech development, fostering a culture of continuous learning and adaptation beyond established practices ensures sustained innovation and competitiveness.
Emphasizing the ongoing refinement of tools and methodologies will not only enhance current projects but also equip teams for future technological advances.